This post is a bit different from my usual content. While it includes a snippet of code, it's designed for a less technical audience, thus the flood of screenshots.
I received an atypical cry for help. A couple is splitting and one of the partners is spamming the other with abusive emails. The receiver created a Gmail filter to trash the messages, but Gmail trash retention policy is 30 days and, in moments of weakness, can't resist clicking in the trash and reading them, which understandably exacerbates the issue.
Given the violent nature of the emails, I didn’t want to risk deleting potential evidence, so my suggestion was to create a process that would:
- Auto-forward the emails from that sender to a lawyer
- Delete those emails and purge them from trash, bypassing Gmail's 30-day retention policy
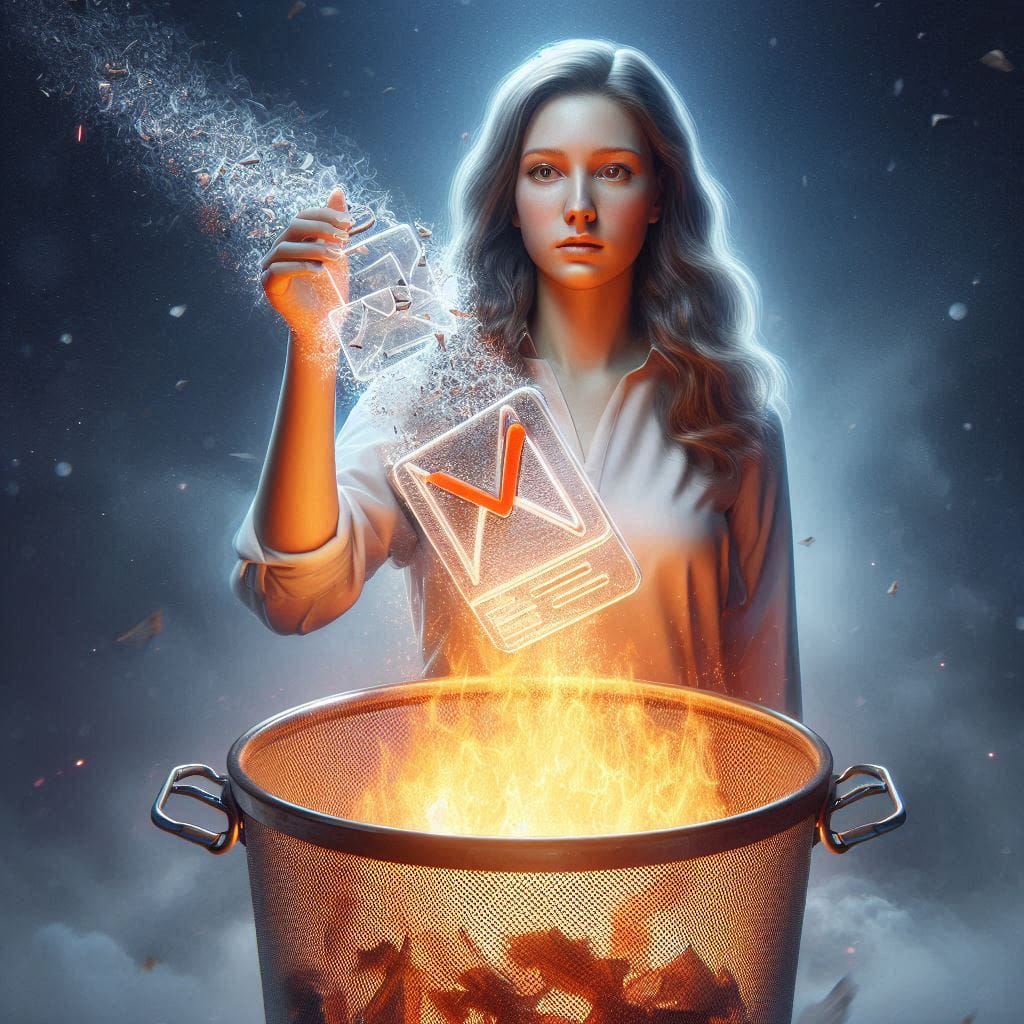
Create a forward address
On this particular case, the forward address will be the lawyer. Go to Gmail settings, select "Forwarding and POP/IMAP" and click on "Add a forwarding address".
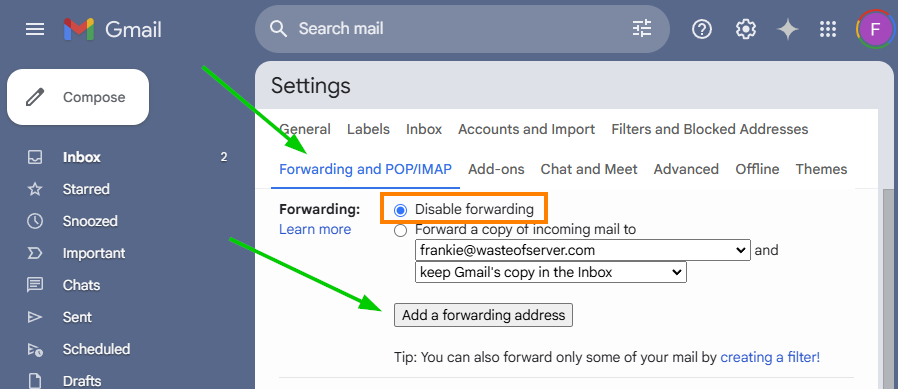
After adding a forward address, Gmail will send a confirmation to that email - your lawyer - asking for permission. As soon as that's granted, you may proceed to the next step. Just remember to keep Forwarding
disabled!
Create a new Filter
Here we will be specifying that all emails that come from [email protected]
will be forwarded to [email protected]
and then sent to the trash.
Go to "Filters and Blocked Addresses" and then click on "Create a new filter".
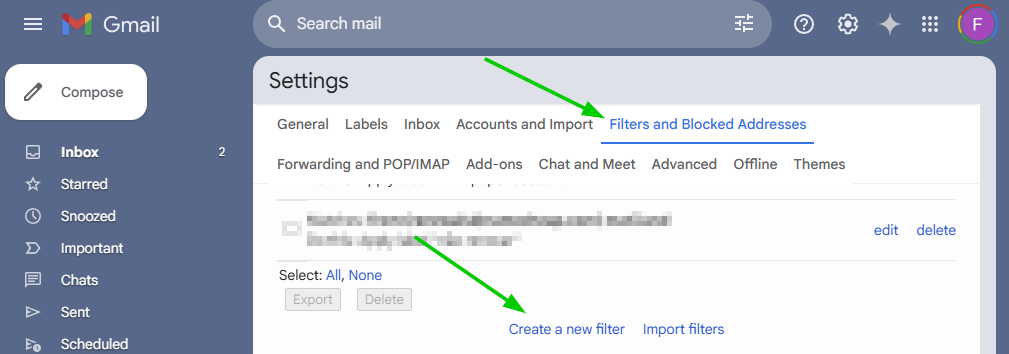
The form for adding filters will open. You want all messages from that specific address to be filtered, so just add "[email protected]" to the filter and choose "Create filter".
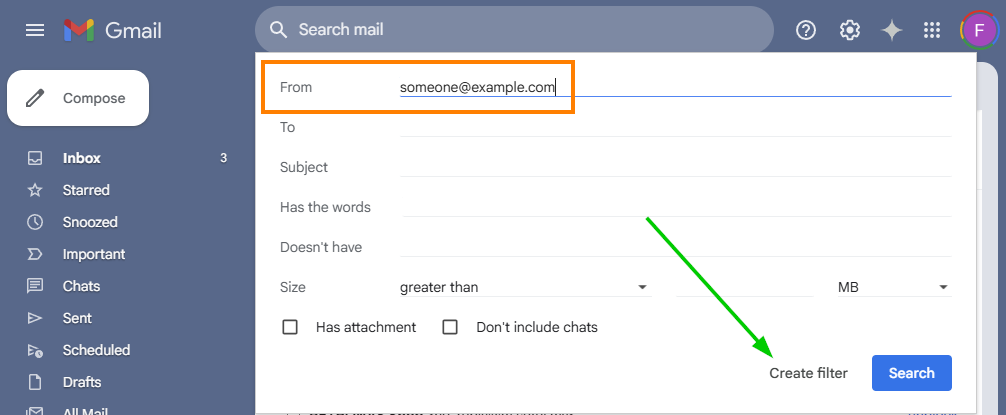
Now you'll have to select exactly what you want the filter to do. On our specific case, we'll forward the email to the lawyer, so tick that box. We also want to delete the email, so also tick that one.
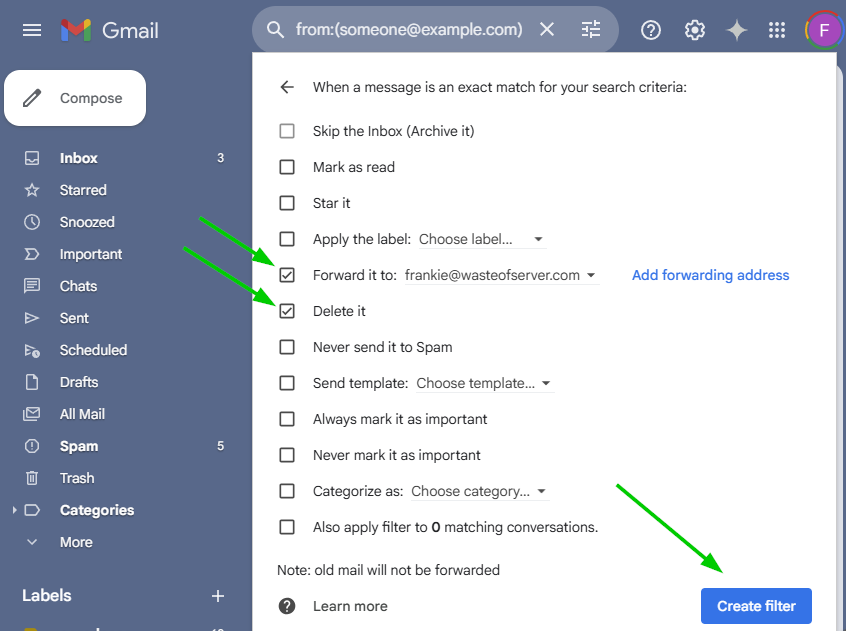
This solves half of the issue and is the most straightforward part of the process. The tricky bit comes next, how to instantly purge those emails from the trash so that we're not tempted to read them. Google Apps Script to the rescue!
Create a Google Apps Script
Google Drive offers a feature that allows you to host and run scripts. While most developers are familiar with this, power users may not be aware of it. For the task at hand, this feature is absolutely perfect.
Head into https://script.google.com/, follow the authentication procedures, if needed, and then click on "New Project".
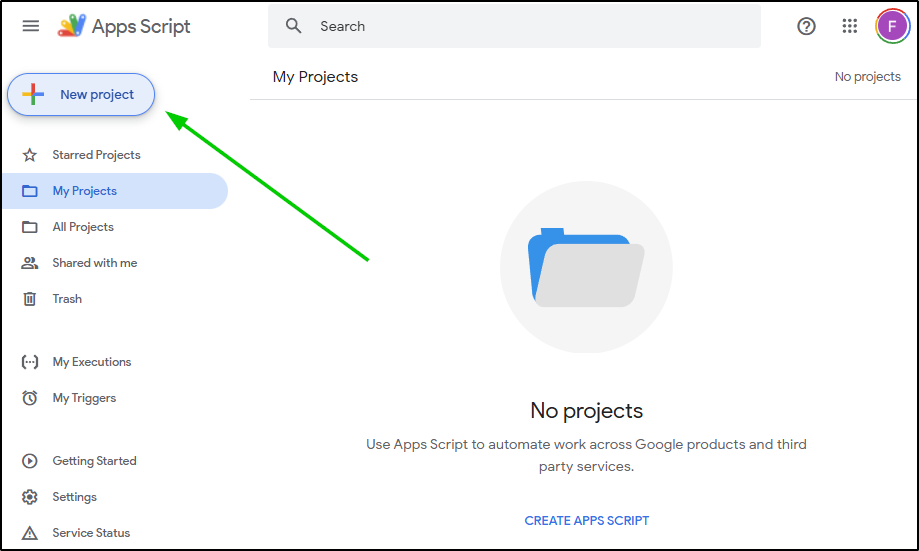
You're now on your project screen. You'll need to interact with Gmail so let's add that service. Click on the big +
next to "Services", look for Gmail API
and add it.
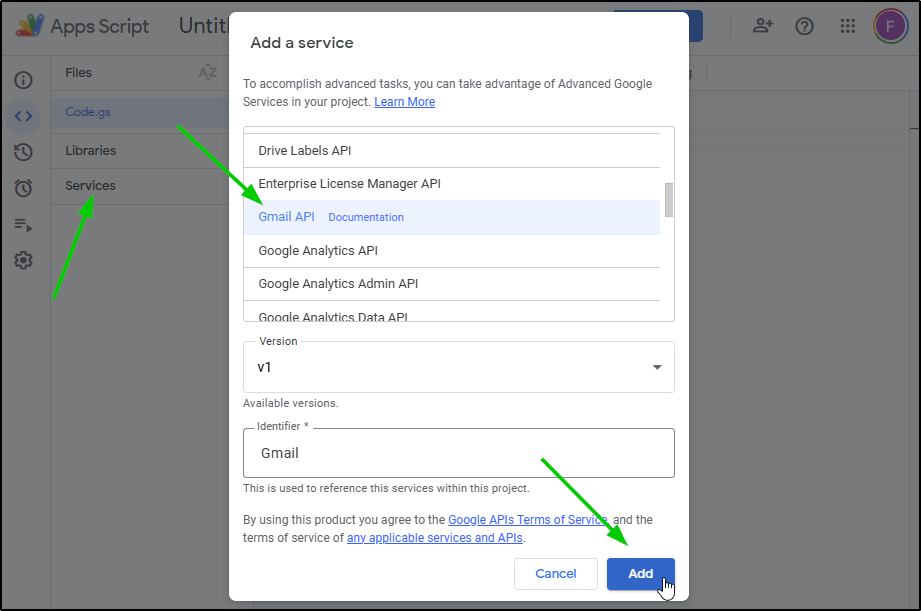
Now, replace the myFunction
with this piece of code. Remember, YOU MUST CHANGE [email protected] to the actual address you want to remove from trash!
function deleteMailsFromTrash() {
var gmailSearchString = `in:trash from:[email protected]`
var threads = GmailApp.search(gmailSearchString);
const n = threads.length;
if (n <= 0) {
Logger.log("No threads matching search string \"%s\"", gmailSearchString);
return
} else {
Logger.log("%s threads matching action **%s**", n, gmailSearchString);
}
for (var i = 0; i < threads.length; i++) {
var thread = threads[i];
Logger.log(`\t Thread# ${i} [ID: ${thread.getId()}]: [message : ${thread.getFirstMessageSubject()}] deleted`);
Gmail.Users.Threads.remove('me', thread.getId());
}
}
This script will look in the trash for emails from [email protected]
and delete them
Your screen should now resemble this. Go ahead and rename "Untitled project" to something more meaningful, like "Purge Specific Mails from Trash". Also change myFunction
to deleteMailsFromTrash
and then hit Run
.
You'll be asked to give permissions to access your Google account.
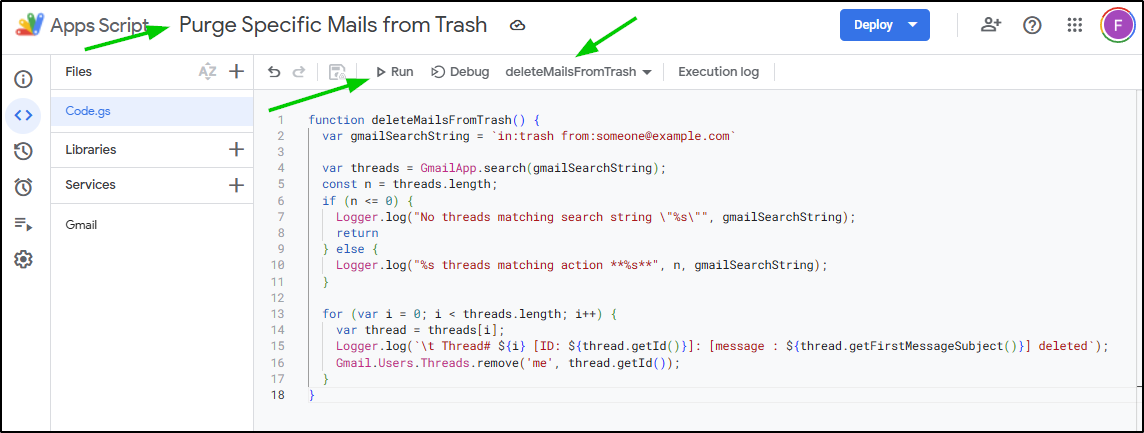
Now you'll get an error! Google hasn't verified this app. While the developer hasn't verified the app, you shouldn't use. In this particular case, you are the developer! That's why I didn't release this solution as a pre-made script. It's safer to have the code running on your side.
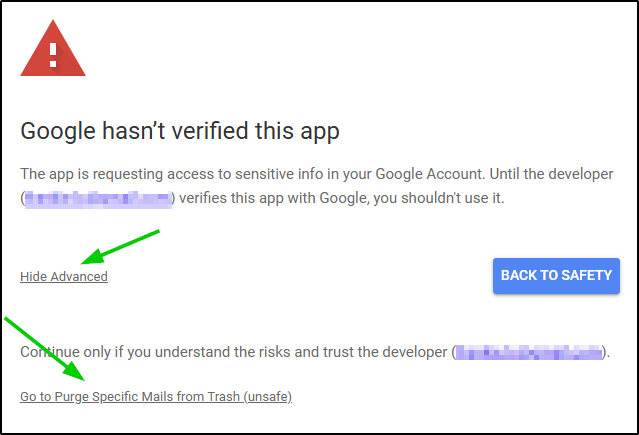
Click on that "Go to Purge Specific Mails from Trash (unsafe)" link and then continue. On your Apps Script window, you'll see the first execution of your script.
On my case, since I don't have email from [email protected]
on the "trash" the program simply prints a "No threads matching search string". On your specific case, you may see that a couple of emails have been deleted! Well done.
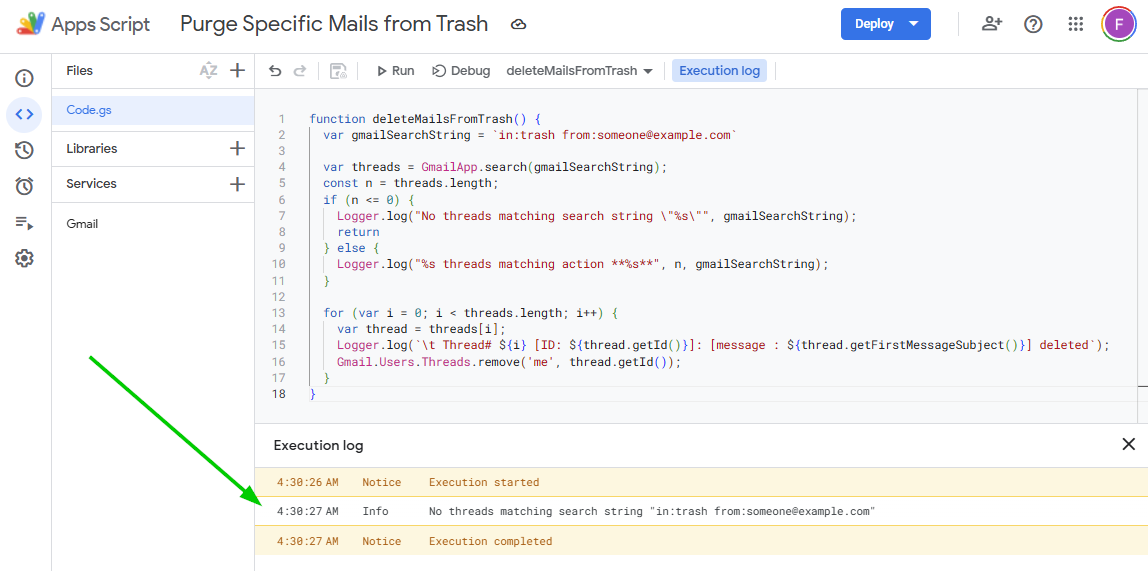
Now everything is working, but we still need to set up a trigger to run the script automatically, ensuring unwanted emails are deleted in a timely manner.
Configure a trigger!
You'll want a time driven trigger. Something that runs on a schedule making sure that the emails that were placed in the trash by the filter you created above are purged before you can get to them.
Click on the clock on the left sidebar, then on the big blue button on the bottom right that says "Add Trigger" and configure the filter as per the image below.
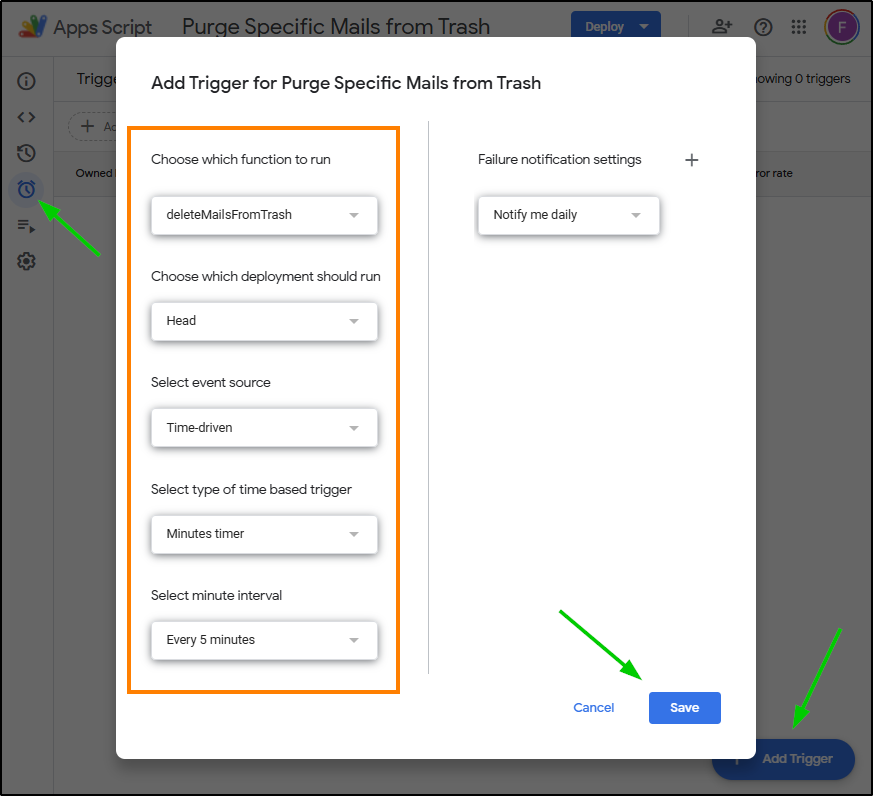
I’ve set this script to run every 5 minutes, but you can adjust the interval to as low as 1 minute if you prefer. Tweak as needed. Setting a longer interval is simply a way to considerate of Google's infrastructure.
Now, to make sure things are working as expected, on the left sidebar, click on executions. You'll see a table with all the times the script has run. As you've just implemented it, there should probably be two to three runs. One manual Type: Editor
from when you manually executed it and then a couple more from the time-driven trigger labeled with Type: Time-Driven
.
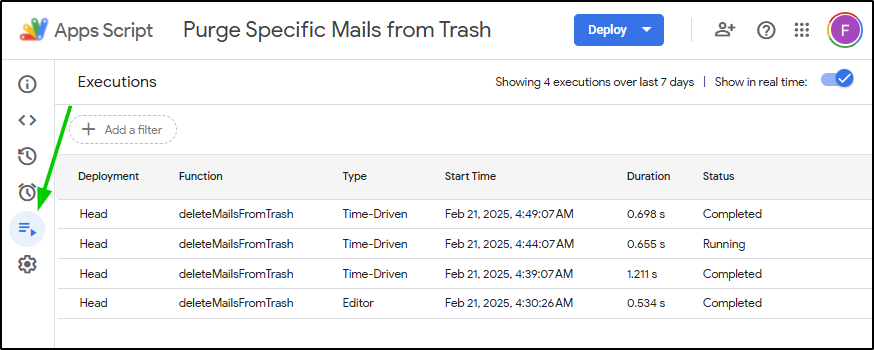
As challenging as it may be to navigate these situations, it’s important to remember that protecting your health is a priority.
While technology can help us minimize harmful distractions, healing takes time and self-compassion. Stay strong, take care of yourself, and don’t hesitate to seek support.
You deserve peace and healing through this process.
I don’t know if you’ve tried rucking before, but it’s something that liberates my mind.
It’s simply walking while carrying weight. Originally a military exercise, rucking is gaining popularity for its benefits to physical health, stability, and mental well-being. Give it a try!
As an Amazon Associate I may earn from qualifying purchases on some links.
If you found this page helpful, please share.